How to use React Native Community Clipboard
Request, Deliver, Revise, Done
Get unlimited UI Design & React Development for a fixed monthly price
50% off your first month
Introduction
Need to read from and write to the device clipboard in your React Native app? Luckily accessing the clipboard is very easy in React Native thanks to a community package called React Native Community Clipboard. Best of all, it works not only on iOS and Android but on Windows and MacOS too.
Installing React Native Community Clipboard
To install React Native Community Clipboard, simply run the following NPM command in your project root:
If you are using Yarn rather than NPM, you can install the package using the following command:
In order for the package to work on iOS, you need to install the associated cocoapods package. Simply run the following command in your project root:
You are now ready to go!
Check you're using the correct package
Just a quick thing you might need to be aware of - the clipboard package used to be a part of the React Native package itself, but has since been removed and taken over and maintained as a community package.
Make sure when you import the Clipboard module your import statement references the community package as follows, rather than from React Native directly:
How to copy to the clipboard with React Native Community Clipboard
First let's create the basic skeleton of our component which we'll use to read and write from the clipboard:
Now we can import the Clipboard API from the package at the top of our component:
Let's add a state value which will store the text value from our text input field:
Next we'll add a function which will take our text state value and copy it to the clipboard:
Now let's add our TextInput and use it to write the value to our state value when it changes using the onTextChange method:
And then we'll add a button below our TextInput which will run our copyToClipboard function when pressed:
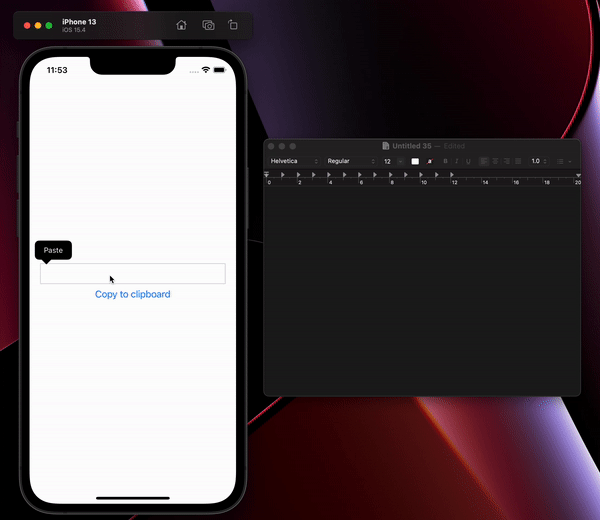
As in the image above, you should now be able to type some text into the text input, click the button, and it should be copied to your clipboard allowing you to paste it elsewhere.
See below for the full code for our copying example:
How to paste from the clipboard with React Native Community Clipboard
Now let's add a method to paste text from our clipboard and set it as our text value, using the getString method. This will read the value from the device's clipboard, and then we use the setText function to write it to our state value:
Then all we need to do is add another button to the component which will run the pasteFromClipboard method:
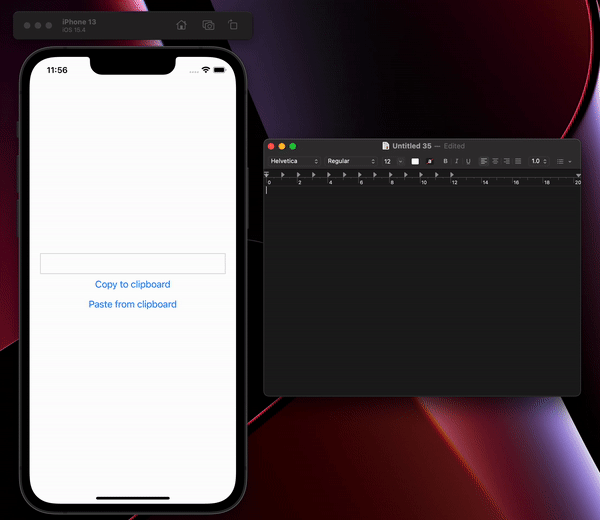
Now you should be able to paste from your clipboard! See the full code below: